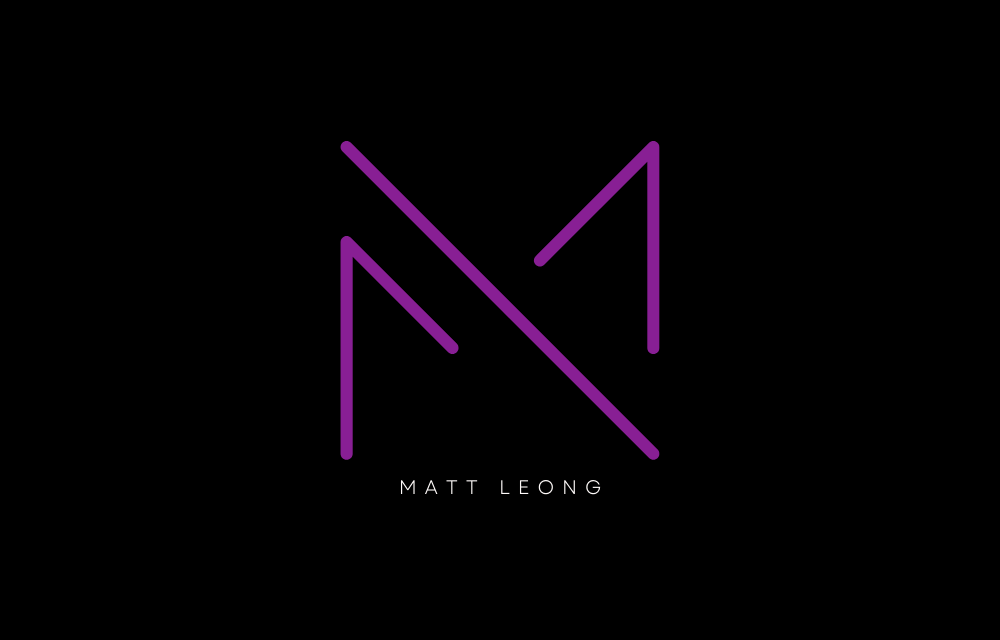
Understanding the Drawbacks of Hooks Returning Components in React
by Matt Leong
React hooks have revolutionized the way we write components, offering a more intuitive and functional approach. However, a common pattern that often emerges is hooks returning components. While it might seem convenient at first , this approach can lead to several issues. Let’s dive into why this is and explore some examples.
Breaking Composition
React is all about component composition – building small, reusable pieces that can be combined in various ways. When a hook returns a component, it obscures this composition, making it harder to understand and reuse the component structure.
Example:
function useGreeting() {
return function Greeting() {
return <h1>Hello, world!</h1>;
};
}
function App() {
const Greeting = useGreeting();
return <Greeting />;
}
Here, useGreeting
returns a Greeting
component. This pattern breaks the clear
separation of concerns and makes it hard to reuse Greeting in different contexts.
Testing Challenges
Testing becomes more complex when hooks return components. You’re no longer testing just the logic or the UI, but a blend of both, which can lead to less effective tests.
Loss of Clarity
When hooks start returning components, it can lead to confusion about where certain functionalities are defined. This loss of clarity makes the code harder to read and maintain.
Performance Concerns
Returning a new component from a hook can lead to performance issues. Each render might create a new instance of the component, leading to unnecessary re- renders and decreased performance.
A Better Approach: Separating Logic and Presentation
Instead of returning components, hooks should be used for managing state and side effects, and components should handle rendering. This separation keeps your code more organized, maintainable, and testable.
Refactored Example:
function useGreeting() {
const greeting = 'Hello, world!';
return greeting;
}
function Greeting() {
const greeting = useGreeting();
return <h1>{greeting}</h1>;
}
function App() {
return <Greeting />;
}
In this refactored example, useGreeting
returns only the data (the greeting
message), and the Greeting
component is responsible for rendering that data.
Stick to Principles
While React’s flexibility is one of its greatest strengths, it’s essential to stick to basic principles such as the separation of concerns. Hooks are a powerful tool for managing logic, but they should not overstep into the realm of rendering components. By keeping our hooks and components distinct, we create more readable, maintainable, and performant React applications.