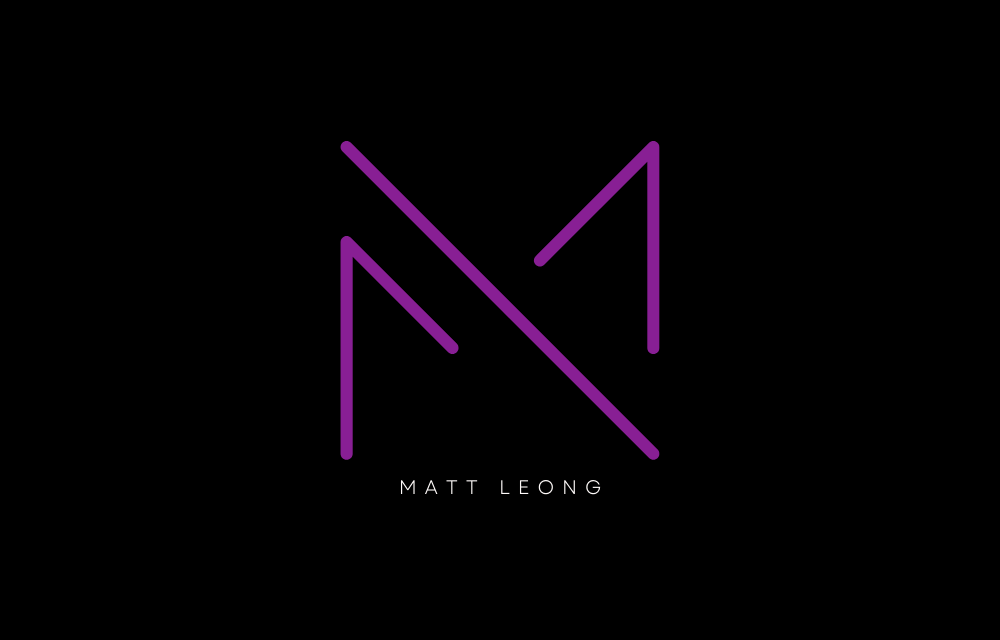
Moving Beyond Prop Drilling
by Matt Leong
Managing data between components in React can be a challenging yet is one of the
most
essential parts of application development. Prop drilling, where data is passed
down through props from parent to child components, is a simple and natural
approach but
can become cumbersome as applications grow. Let’s first look at how prop
drilling works, particularly with useState
, and then explore more scalable alternatives.
Understanding Prop Drilling with useState
Prop drilling is like passing a message down a line of people. It works well
in small scenarios but becomes inefficient as the line grows. In React, this
often involves using useState
in a parent component and passing the state down
through props.
Example of Prop Drilling with useState
Consider a scenario where you have a user’s data that needs to be shared across multiple components:
// In the Parent Component
function ParentComponent() {
const [user, setUser] = useState(null);
return <ChildComponent user={user} setUser={setUser} />;
}
// In the Child Component
function ChildComponent({ user, setUser }) {
// Use user and setUser in this component
return <GrandchildComponent user={user} setUser={setUser} />;
}
// In the Grandchild Component
function GrandchildComponent({ user, setUser }) {
// Use user and setUser in this component
}
In this example, the user state is defined in ParentComponent
and needs to be
passed down to ChildComponent
and GrandchildComponent
. Each component requires
both the user data and the setUser function to update it, leading to prop drilling.
The Limitations of Prop Drilling
This approach, while straightforward, has its downsides:
As more components are added to the hierarchy, managing and tracking state through props becomes more challenging.
Maintenance Becomes Difficult: Updating state logic or adding new state variables requires changes at multiple levels.
Performance Concerns: Passing props unnecessarily through components can lead to inefficient rendering.
Embracing Jotai for Global State Sharing
To address these limitations, Jotai offers a simpler and more effective state management solution through its atom-based system, allowing global state sharing across components without the hassle of prop drilling.
The Global Sharing of userAtom
By defining a userAtom
, you create a globally accessible piece of state:
import { atom } from 'jotai';
// Initialize a globally accessible user atom
const userAtom = atom(null);
Any component can then interact with this atom directly:
import { useAtom } from 'jotai';
function UserProfile() {
const [user, setUser] = useAtom(userAtom);
// Access or update user data directly
}
function UserLogin() {
const [, setUser] = useAtom(userAtom);
// Update user state upon login
}
Transitioning from traditional prop drilling to using Jotai streamlines state management in React applications, especially for larger, more complex architectures. By leveraging atoms, Jotai provides an intuitive and efficient way to handle shared state across components, making your development process smoother and your applications more scalable.